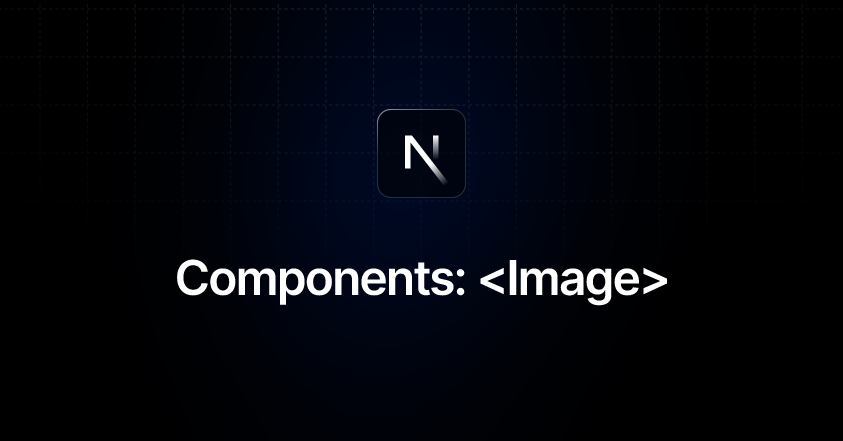
TLDR
- Use
next/image
for large images (>40x40px) that need optimization, responsive behavior, or lazy loading - Don't use it for SVGs, tiny icons, or decorative elements
- Key features:
<Image src="/image.jpg" alt="Description" width={800} // Required to prevent layout shift height={600} // Required to prevent layout shift priority // For above-the-fold images fill // For parent-based sizing sizes="(max-width: 768px) 100vw, 50vw" // For responsive images />
- Always wrap
fill
mode images in arelative
parent with defined dimensions - Use
priority
for hero images and critical above-the-fold content - Handle responsive images with aspect ratio containers and proper
sizes
prop
As web developers, we often struggle with image optimization and responsive design. Next.js provides a powerful next/image
component that helps solve these challenges, but it can be tricky to understand when and how to use it effectively. In this guide, I'll break down the best practices and common patterns for using the Next.js Image component.
Table of Contents
When to Use next/image
The next/image
component is powerful but isn't always the right choice. Here's when you should and shouldn't use it:
Good Use Cases
1. Hero Images / Banner Images
function Hero() { return ( <Image src="/hero.jpg" alt="Hero banner" width={1920} height={1080} priority // Load immediately as it's above the fold className="w-full h-auto" // Responsive sizing /> ); }
2. Product Images
function ProductCard({ product }) { return ( <div className="relative aspect-square"> <Image src={product.imageUrl} alt={product.name} fill // Use fill for parent-based sizing sizes="(max-width: 768px) 100vw, (max-width: 1200px) 50vw, 33vw" className="object-cover" // Maintain aspect ratio /> </div> ); }
3. Profile Pictures
function Avatar({ user }) { return ( <div className="relative w-10 h-10 rounded-full overflow-hidden"> <Image src={user.avatar} alt={`${user.name}'s avatar`} fill sizes="40px" // Fixed size across all breakpoints className="object-cover" /> </div> ); }
When Not to Use
Don't use next/image
for:
- SVG icons
- Small decorative images
- Images that don't need optimization
- Images smaller than 40x40 pixels
Responsive Patterns
1. Fixed Aspect Ratio
function ResponsiveCard() { return ( <div className="relative aspect-video w-full"> <Image src="/feature.jpg" alt="Feature" fill sizes="(max-width: 768px) 100vw, 50vw" className="object-cover" /> </div> ); }
2. Grid Layout
function ImageGrid() { return ( <div className="grid grid-cols-1 md:grid-cols-2 lg:grid-cols-3 gap-4"> {images.map((image) => ( <div key={image.id} className="relative aspect-square"> <Image src={image.url} alt={image.alt} fill sizes="(max-width: 768px) 100vw, (max-width: 1200px) 50vw, 33vw" className="object-cover" /> </div> ))} </div> ); }
3. Art-directed Images
function ArtDirectedHero() { return ( <> {/* Mobile Image */} <Image src="/hero-mobile.jpg" alt="Hero" width={640} height={640} className="block md:hidden w-full h-auto" /> {/* Desktop Image */} <Image src="/hero-desktop.jpg" alt="Hero" width={1920} height={1080} className="hidden md:block w-full h-auto" /> </> ); }
Dynamic Images
1. With Blur Placeholder
function ProductImage({ product }) { return ( <Image src={product.image} alt={product.name} width={400} height={400} placeholder="blur" blurDataURL={product.blurHash} // Base64 or blur hash className="w-full h-auto" /> ); }
2. With Loading State
function LazyLoadedImage({ src, alt }) { return ( <div className="relative aspect-square bg-gray-100"> <Image src={src} alt={alt} fill className="object-cover transition-opacity duration-300" loading="lazy" sizes="(max-width: 768px) 100vw, (max-width: 1200px) 50vw, 33vw" /> </div> ); }
Layout Patterns
1. Magazine Layout
function MagazineLayout() { return ( <div className="grid grid-cols-12 gap-4"> {/* Featured Image */} <div className="col-span-12 md:col-span-8 relative aspect-[16/9]"> <Image src="/featured.jpg" alt="Featured" fill priority className="object-cover" sizes="(max-width: 768px) 100vw, 66vw" /> </div> {/* Sidebar Images */} <div className="col-span-12 md:col-span-4 space-y-4"> {sideImages.map((img) => ( <div key={img.id} className="relative aspect-square"> <Image src={img.url} alt={img.alt} fill className="object-cover" sizes="(max-width: 768px) 100vw, 33vw" /> </div> ))} </div> </div> ); }
2. Masonry Layout
function MasonryGrid() { return ( <div className="columns-1 md:columns-2 lg:columns-3 gap-4"> {images.map((image) => ( <div key={image.id} className="relative mb-4 break-inside-avoid"> <Image src={image.url} alt={image.alt} width={image.width} height={image.height} className="w-full h-auto" /> </div> ))} </div> ); }
Best Practices
1. Image Component with Error Handling
function SafeImage({ src, alt, ...props }) { const [error, setError] = useState(false); if (error) { return ( <div className="bg-gray-100 flex items-center justify-center"> <span>Image not available</span> </div> ); } return ( <Image src={src} alt={alt} onError={() => setError(true)} {...props} /> ); }
2. Optimized Loading Strategy
function GalleryImage({ priority, ...props }) { return ( <div className="relative aspect-square"> <Image {...props} fill priority={priority} loading={priority ? undefined : 'lazy'} className="object-cover" sizes="(max-width: 768px) 100vw, (max-width: 1200px) 50vw, 33vw" /> </div> ); }
Key Takeaways
-
Use
next/image
for:- Large images that need optimization
- Images that benefit from lazy loading
- Responsive images
- Critical above-the-fold images (with priority)
-
Performance Tips:
- Use
priority
for above-the-fold images - Provide correct
sizes
attribute - Use blur placeholders for better UX
- Consider art direction for different viewports
- Optimize image dimensions at build time
- Use
-
Layout Considerations:
- Use aspect ratio containers
- Consider responsive designs
- Use appropriate sizing strategies
- Handle loading states
- Provide fallbacks
The fixed width and height requirement in next/image
might seem limiting at first, but it's actually a feature that prevents layout shift during loading. By combining these requirements with CSS, you can create fully responsive images while maintaining optimal performance.
Conclusion
The Next.js Image component is a powerful tool for optimizing images in your web applications. By following these patterns and best practices, you can ensure your images are performant, responsive, and provide a great user experience across all devices.
Remember, while next/image
offers many benefits, it's not always the right choice. Consider your specific use case and choose the appropriate solution for your needs.
This guide is based on Next.js 13+ and reflects current best practices as of 2024.