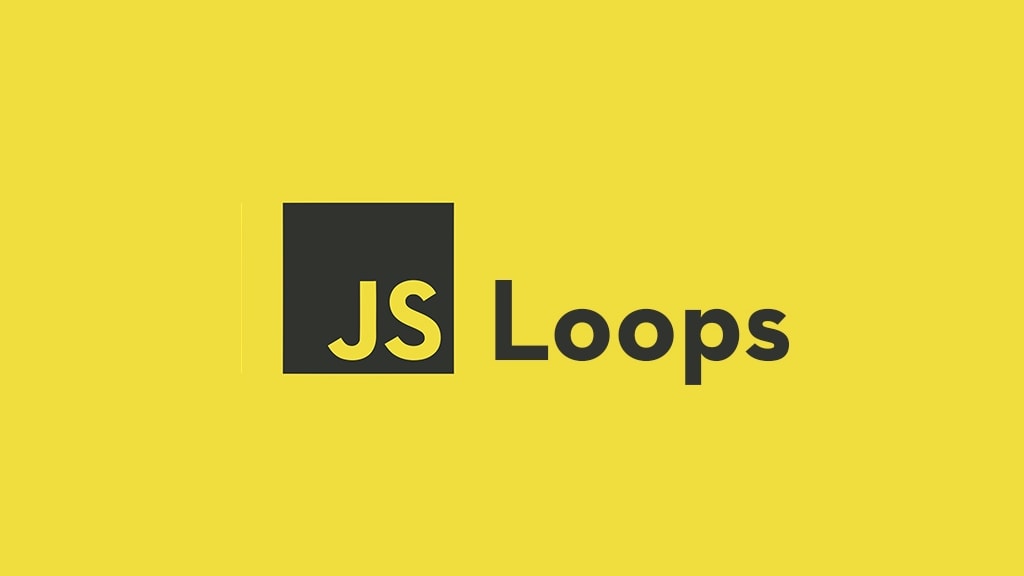
In the world of JavaScript performance optimization, choosing the right looping construct can make a significant difference in your application's efficiency. Today, we'll dive deep into a performance comparison of different JavaScript looping methods, with benchmark data from major browsers including Chrome 85, Microsoft Edge, and Firefox 80.
The Benchmark Setup
Benchmark lab: https://jsben.ch/EAlKw (Update in 2024, the lab looks like it has problems. 😥)
Our test environment consisted of:
- Browsers: Chrome 85, Microsoft Edge, Firefox 80
- Array size: 1,000,000 elements
- Operation: Simple assignment to a variable
- Measurement: Relative performance (higher percentage = better performance)
Test initialization code:
var arr = Array(1000000).fill(null) var dosmth
Performance Results
The performance patterns show remarkable consistency across browsers, with some interesting variations:
-
Basic
for
loop with uncached lengthfor (let i = 0; i < arr.length; i++) { dosmth = arr[i] }
- Consistently top performer in Chrome and Edge
- Strong showing in Firefox, though slightly behind cached version
-
Basic
for
loop with cached lengthlet len = arr.length for (let i = 0; i < len; i++) { dosmth = arr[i] }
- Nearly identical performance to uncached version in all browsers
- Takes the lead in Firefox benchmarks
-
while
loop variantslet i = 0 const len = arr.length while (i < len) { dosmth = arr[i] ++i }
- Strong performers across all browsers
- Particularly efficient in Edge, showing identical performance to for loops
- Both cached and uncached versions show similar results
-
for...of
loopfor (let val of arr) { dosmth = val }
- Consistent mid-tier performance across browsers
- Modern and clean syntax
- Good balance of readability and performance
-
forEach
arr.forEach(el => (dosmth = el))
- Performance varies significantly between browsers
- Notably better performance in Firefox compared to Chrome and Edge
- Function call overhead impacts performance
-
map
arr.map(el => (dosmth = el))
- Consistently the slowest option across all browsers
- Creates unnecessary array overhead
- Best avoided for simple iterations
Key Insights
-
Traditional is Still King
- Classic
for
loops remain the performance champions across all browsers - Modern JS engines heavily optimize these patterns
- The simplest approach proves to be the most efficient
- Classic
-
Length Caching Myth
- Caching array length shows minimal impact in modern browsers
- Modern JavaScript engines optimize this internally
- Choose based on code readability rather than perceived performance benefits
-
Modern vs. Traditional
- Modern methods prioritize readability over raw performance
for...of
offers a good compromise across all browsers- Browser optimization strategies vary, but patterns remain consistent
-
Functional Methods Trade-offs
- Performance impact varies significantly between browsers
- Firefox shows notably better optimization for functional methods
- Still generally slower than traditional loops
Recommendations
-
For Performance-Critical Code:
// Best cross-browser performance for (let i = 0; i < arr.length; i++) { dosmth = arr[i] }
-
For Maintenance and Readability:
// Good balance for (let val of arr) { dosmth = val }
-
For Functional Programming Style:
// When performance isn't critical arr.forEach(el => (dosmth = el))
Consider the Context:
- For small arrays (< 1000 elements), the performance difference is negligible
- Modern hardware can handle most scenarios efficiently
- Code readability often trumps micro-optimizations
- Browser-specific optimizations exist but don't significantly impact general usage patterns
Conclusion
While traditional for
loops remain the performance champions across all major browsers, the choice of looping construct should depend on your specific needs. For most applications, the readability and maintainability benefits of modern methods outweigh their performance costs. Only optimize with traditional loops when dealing with performance-critical operations or large datasets.
Here's a practical decision tree:
- Need maximum performance? → Use traditional
for
loop - Want clean, readable code? → Use
for...of
- Working with functional programming patterns? → Use
forEach
ormap
- Small array or non-critical section? → Use whatever is most readable
Remember: premature optimization is the root of all evil. Choose the most readable and maintainable option first, then optimize if profiling shows it's necessary.